Progress 进度条
#
例子#
基本用法import { Window, ProgressBar, Grid } from 'ave-ui';
export function main(window: Window) { const progressBar = new ProgressBar(window); progressBar.SetMaximum(100).SetAnimation(true); progressBar.SetValue(75);
const container = getControlDemoContainer(window); container.ControlAdd(progressBar).SetGrid(1, 1); window.SetContent(container);}
function getControlDemoContainer(window: Window, count = 1) { const container = new Grid(window); container.ColAddSlice(1); container.ColAddDpx(...Array.from<number>({ length: count }).fill(240)); container.ColAddSlice(1);
container.RowAddSlice(1); container.RowAddDpx(...Array.from<number>({ length: count }).fill(32)); container.RowAddSlice(1); return container;}
在这个例子中,我们演示了进度条的基本用法:
其中SetAnimation
设置的动画是进度条中光影的流动。
#
APIexport interface IProgressBar extends IControl { SetMaximum(value: number): ProgressBar; GetMaximum(): number;
SetValue(value: number): ProgressBar; GetValue(): number;
SetAnimation(enableAnimation: boolean): ProgressBar;
// 是否启用了动画效果 GetAnimation(): boolean;}
#
状态通过 SetState
可以设置进度条状态:
import { Window, ProgressBar, Grid, ProgressBarState } from 'ave-ui';
export function main(window: Window) { const progressBar = new ProgressBar(window); progressBar.SetMaximum(100).SetAnimation(true); progressBar.SetValue(75); progressBar.SetState(ProgressBarState.Normal);
const container = getControlDemoContainer(window); container.ControlAdd(progressBar).SetGrid(1, 1); window.SetContent(container);}
- Normal
- Paused
- Error
- Pulse
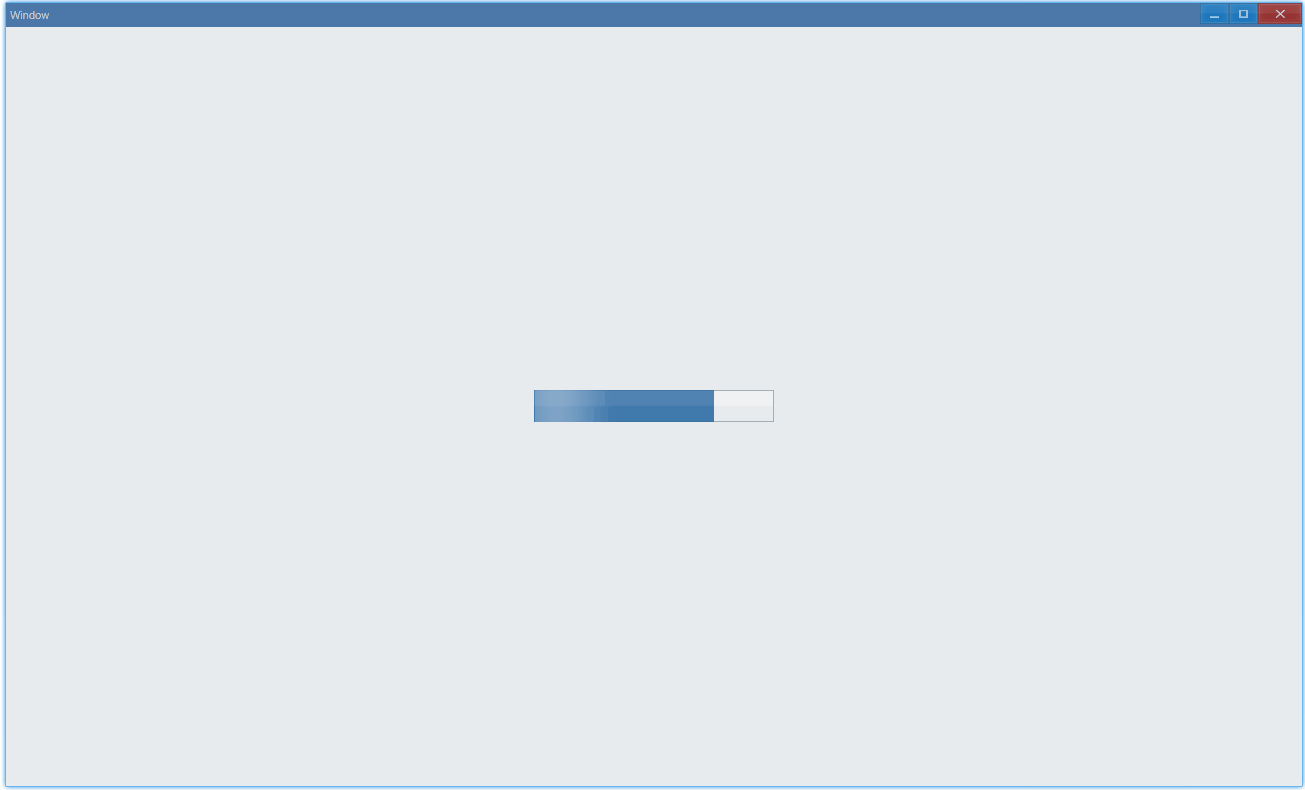
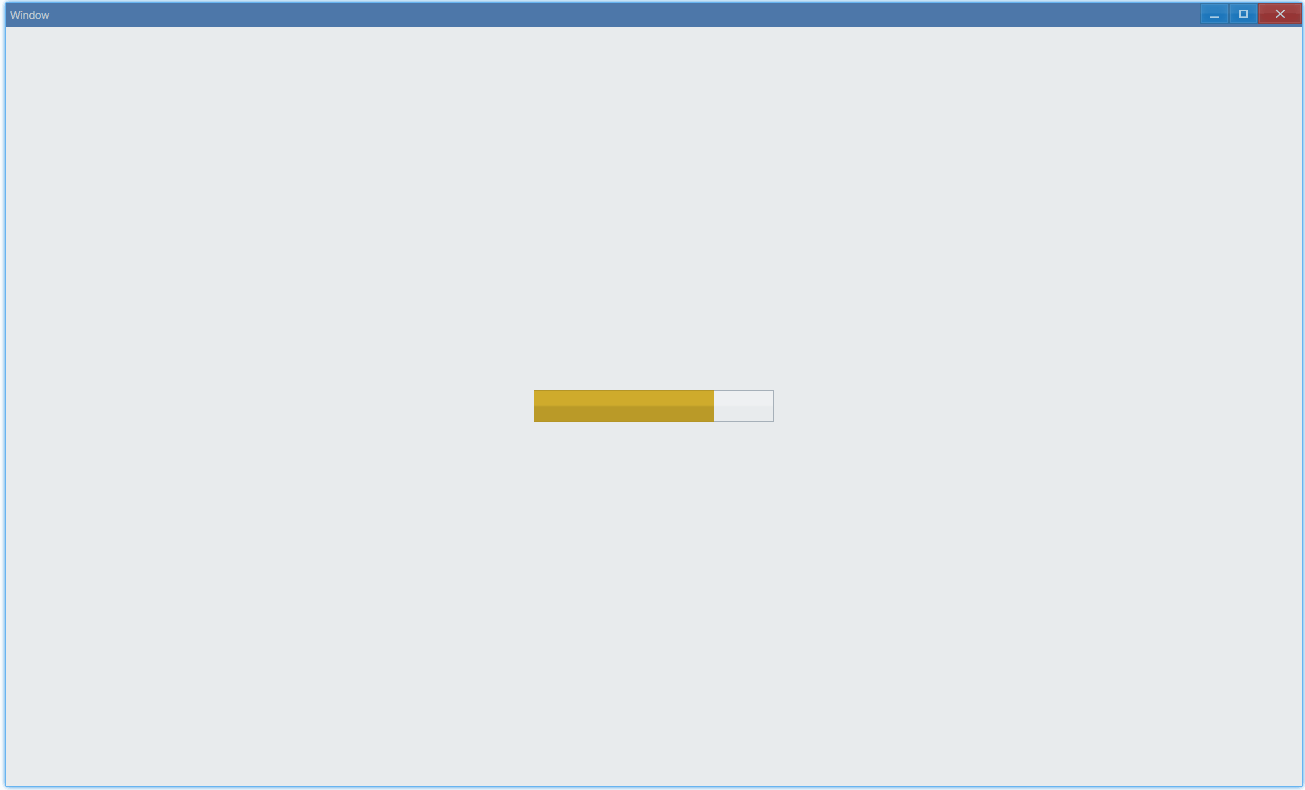
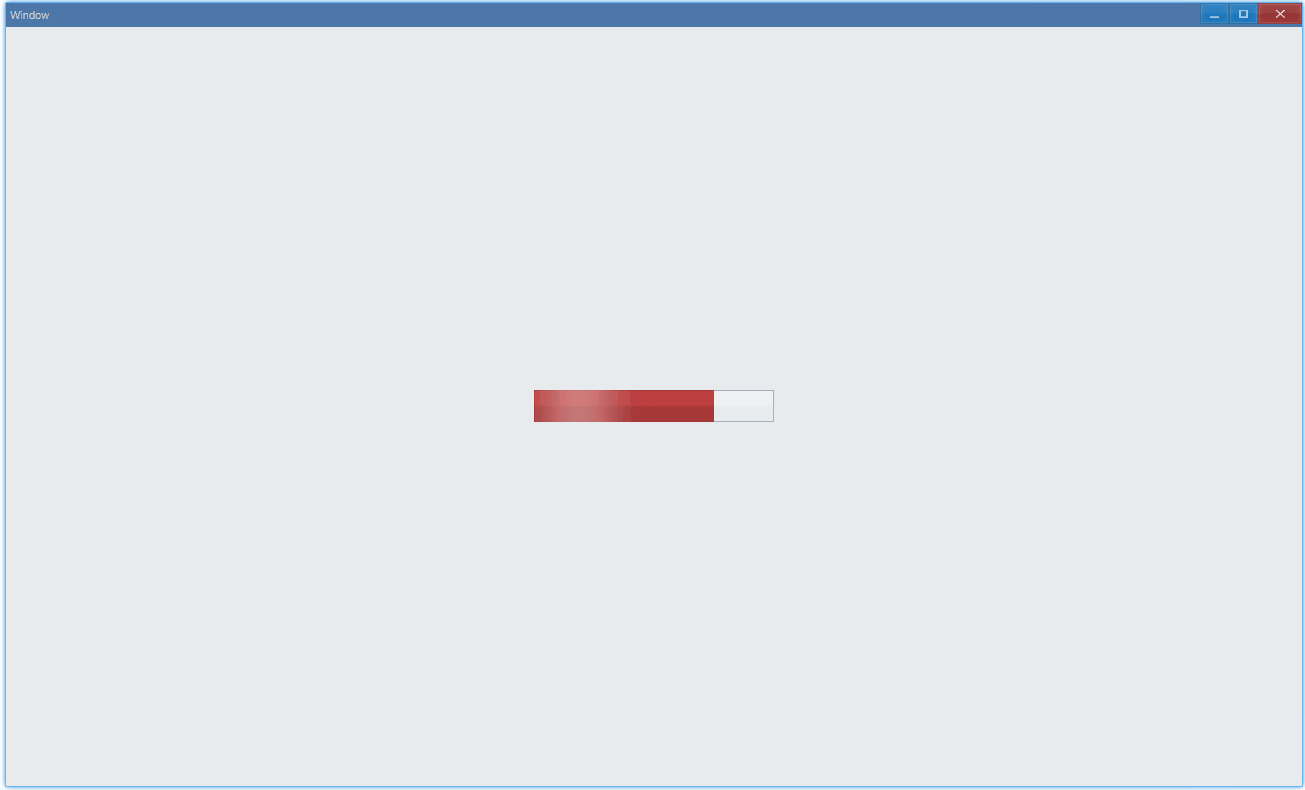
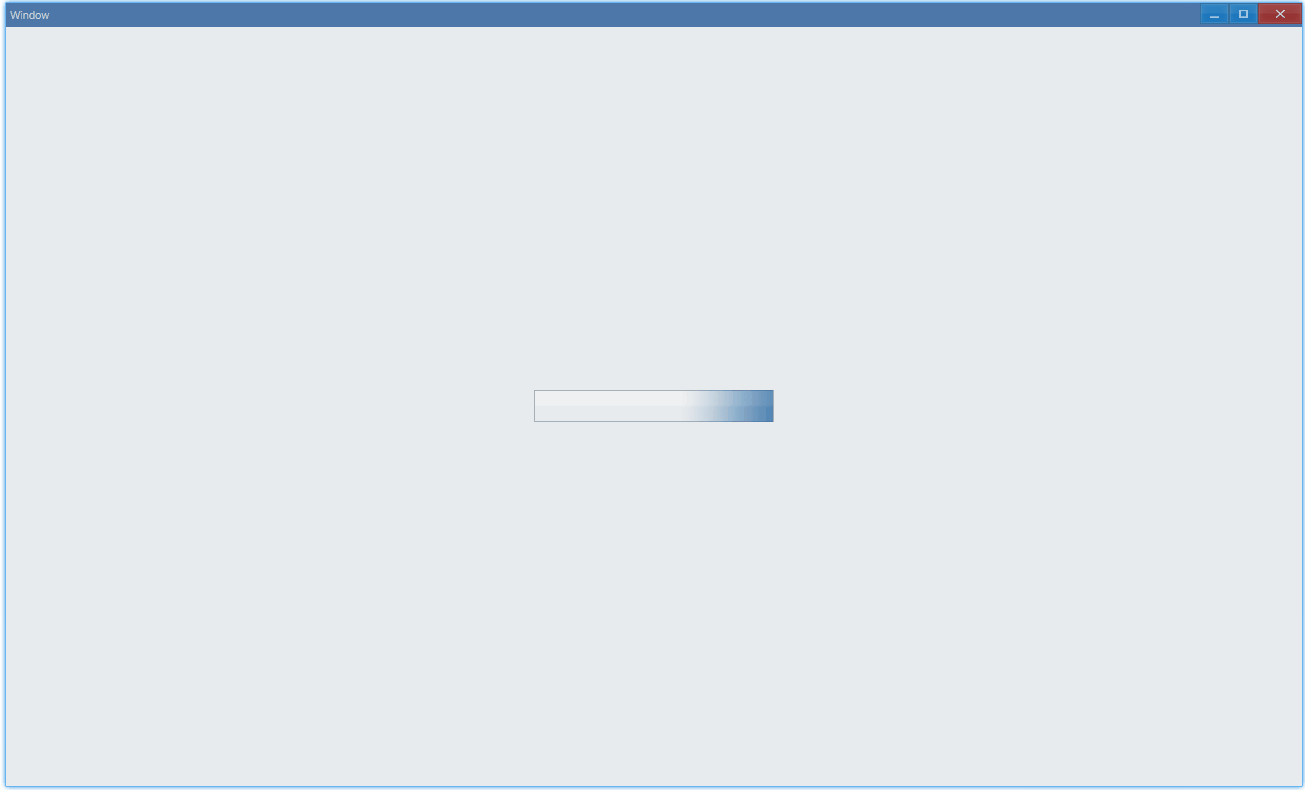
#
APIexport interface IProgressBar extends IControl { SetState(state: ProgressBarState): ProgressBar; GetState(): ProgressBarState;}
export enum ProgressBarState { Normal, Paused, Error, Pulse, None,}
#
步长(每次增加多少)import { Window, ProgressBar, Grid } from 'ave-ui';
export function main(window: Window) { const progressBar = new ProgressBar(window); progressBar.SetMaximum(100).SetAnimation(true);
// 从0开始增长 progressBar.SetValue(0);
// 每次增加1 progressBar.SetStep(1);
const container = getControlDemoContainer(window); container.ControlAdd(progressBar).SetGrid(1, 1); window.SetContent(container);
const id = setInterval(() => { if (progressBar.GetValue() < 100) { // 每隔100ms就增长一次 progressBar.Step(); } else { clearInterval(id); } }, 100);}
这个例子演示了怎样实现通常我们看到的“进度条不断增长”的效果:
#
APIexport interface IProgressBar extends IControl { SetStep(value: number): ProgressBar; GetStep(): number; Step(): ProgressBar;}